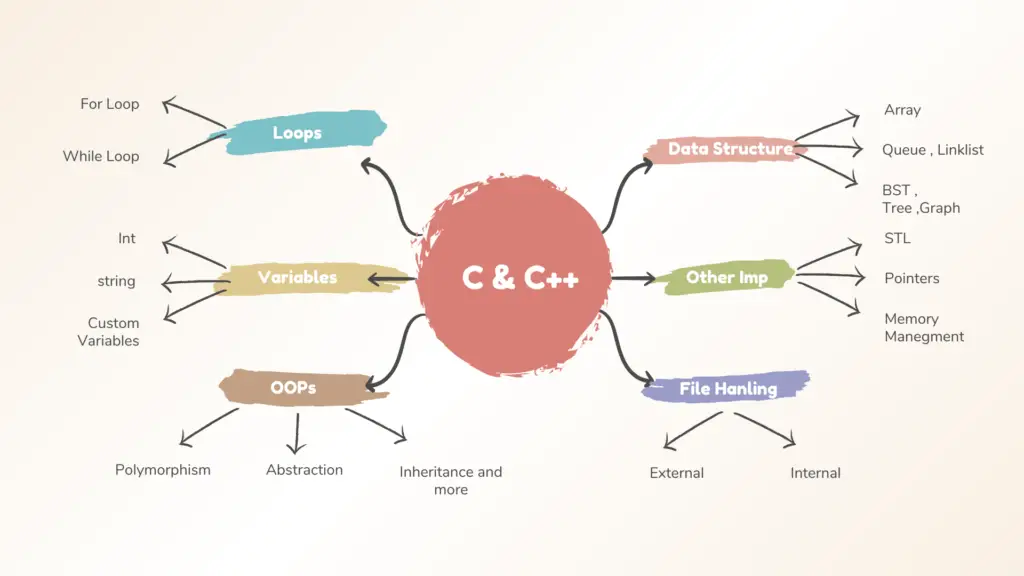
Basic
C++
- Introduction
- Looping
- Data Types
- Variables and more
Intermediate
C++
- Function
- Pointer
- Data Structure :Linklist,
- ,Tree, Graph
Advanced
C++
- File Handling
- STL (Standard Template Library)
- Memory Management
Heading | Reference Link |
---|---|
1. Introduction to C and C++ Programming | Link |
2. Setting Up the Development Environment | Link |
3. Basic Syntax and Data Types | Link |
4. Control Flow and Decision-Making | Link |
5. Functions and Modules | Link |
6. Pointers and Memory Management | Link |
7. Object-Oriented Programming in C++ | Link |
8. File Handling | Link |
9. Exception Handling in C++ | Link |
10. Standard Template Library (STL) | Link |
11. Debugging and Troubleshooting | Link |
12. Best Practices and Coding Tips | Link |
13. Common Use Cases for C and C++ | Link |
14. Recommended Books and Resources | Link |
30 Days Full C and Cpp Programming Plan:
- Day 1: Introduction to C programming language
- Basic syntax and structure
- Data types and variables
- Input and output operations
- Day 2: Control flow and decision making
- Conditional statements (if-else, switch)
- Loops (for, while, do-while)
- Day 3: Functions and modular programming
- Function declaration and definition
- Passing arguments to functions
- Return values and recursion
- Day 4: Arrays and strings
- Declaration and initialization
- Accessing array elements
- String manipulation functions
- Day 5: Pointers and memory management
- Understanding pointers
- Pointers and arrays
- Dynamic memory allocation
- Day 6: Structures and unions
- Creating and accessing structures
- Nested structures and arrays of structures
- Unions and their applications
- Day 7: File handling
- Opening and closing files
- Reading from and writing to files
- File positioning and error handling
- Day 8: Introduction to C++ programming language
- Evolution and features of C++
- Object-oriented programming concepts
- Day 9: Classes and objects
- Class declaration and definition
- Access specifiers (public, private, protected)
- Member functions and data members
- Day 10: Constructors and destructors
- Default constructor
- Parameterized constructor
- Destructor and memory deallocation
- Day 11: Inheritance and polymorphism
- Single inheritance
- Multiple inheritance
- Polymorphism and function overriding
- Day 12: Operator overloading
- Overloading arithmetic and relational operators
- Overloading assignment operator
- Friend functions and operator overloading
- Day 13: Exception handling
- Try-catch blocks
- Throwing and catching exceptions
- Exception handling hierarchy
- Day 14: Templates and generic programming
- Function templates
- Class templates
- Template specialization
- Day 15: Standard Template Library (STL)
- Containers (vector, list, stack, queue)
- Algorithms (sorting, searching, etc.)
- Iterators and function objects
- Day 16-30: Advanced topics and projects
- Advanced data structures
- Multithreading and concurrency
- GUI programming
- Developing a small-scale project
Day 1: Introduction to C programming language
On the first day, we will dive into the basics of the C programming language. You’ll learn about the syntax and structure of C programs, including how to write your first “Hello, World!” program. Understanding data types, variables, and basic input and output operations will also be covered.
Day 2: Control flow and decision making
Day 2 focuses on control flow and decision making in C. You’ll explore conditional statements like if-else and switch, which allow you to execute different blocks of code based on specific conditions. Additionally, you’ll learn about loops such as for, while, and do-while, enabling you to repeat code execution until certain conditions are met.
Day 3: Functions and modular programming
Modular programming is essential for writing maintainable and reusable code. On day 3, you’ll delve into functions in C. You’ll discover how to declare and define functions, pass arguments to them, and handle return values. Recursion, a powerful technique where a function calls itself, will also be introduced.
Day 4: Arrays and strings
Arrays and strings are fundamental data structures in C. This day will cover the declaration, initialization, and accessing of array elements. You’ll also explore string manipulation functions, enabling you to perform common operations on character arrays.
Day 5: Pointers and memory management
Pointers are a key concept in C programming. Day 5 will demystify pointers and their importance in memory management. You’ll learn how to declare and use pointers, understand the relationship between pointers and arrays, and explore dynamic memory allocation.
Day 6: Structures and unions
Structures and unions allow you to create complex data types in C. On this day, you’ll discover how to define and access structures. Nested structures and arrays of structures will also be covered. Additionally, you’ll learn about unions and their applications.
Day 7: File handling
Working with files is essential for many programs. Day 7 introduces file handling in C, including opening and closing files, reading from and writing to files, and handling errors and file positioning. You’ll gain the skills to create programs that can interact with external files.
Day 8: Introduction to C++ programming language
On day 8, you’ll shift your focus to C++ programming language, an extension of C. You’ll explore the evolution of C++ and its key features. Object-oriented programming concepts, such as classes and objects, will be introduced.
Day 9: Classes and objects
Classes are the building blocks of object-oriented programming. Day 9 will cover class declaration, definition, and member functions. Access specifiers like public, private, and protected will be explained, along with their significance in encapsulation.
Day 10: Constructors and destructors
Constructors and destructors are essential for initializing and releasing resources in C++. You’ll learn about default constructors, parameterized constructors, and destructors. Memory deallocation and best practices for resource management will also be discussed.
Day 11: Inheritance and polymorphism
Inheritance allows you to create new classes based on existing ones. Day 11 focuses on single and multiple inheritance in C++, enabling you to build hierarchies of related classes. Polymorphism, achieved through function overriding, will be explored.
Day 12: Operator overloading
Operator overloading allows you to redefine the behavior of operators in C++. On this day, you’ll learn how to overload arithmetic and relational operators. The assignment operator will also be covered, along with the concept of friend functions.
Day 13: Exception handling
Exception handling is crucial for writing robust and fault-tolerant code. Day 13 will introduce you to try-catch blocks, which enable you to handle exceptions gracefully. You’ll learn how to throw and catch exceptions and understand the exception handling hierarchy.
Day 14: Templates and generic programming
Templates allow you to write generic code that works with multiple data types. On this day, you’ll explore function templates### Day 14: Templates and generic programming
Templates allow you to write generic code that works with multiple data types. On this day, you’ll explore function templates, which enable you to create functions that can operate on different data types without duplicating code. Class templates, which allow you to create generic classes, will also be covered. Additionally, you’ll learn about template specialization, which allows you to provide specialized implementations for specific data types.
Day 15: Standard Template Library (STL)
The Standard Template Library (STL) is a powerful library that provides a collection of generic algorithms and data structures. On this day, you’ll dive into the STL and its various components. You’ll learn about containers such as vectors, lists, stacks, and queues, which provide efficient data storage and retrieval. Additionally, you’ll explore algorithms for sorting, searching, and manipulating data. Iterators, which provide a way to traverse elements in a container, and function objects, which allow you to encapsulate functions, will also be covered.
Day 16-30: Advanced topics and projects
In the remaining days of the 30-day road map, you’ll explore more advanced topics and undertake small-scale projects to reinforce your learning. Advanced data structures such as linked lists, trees, and graphs will be covered. You’ll also delve into multithreading and concurrency, allowing your programs to execute multiple tasks simultaneously. Additionally, you’ll learn about GUI programming using frameworks like Qt, enabling you to create graphical user interfaces for your applications. Finally, you’ll have the opportunity to work on a small-scale project, applying the concepts and techniques you’ve learned throughout the road map.
While it’s not strictly necessary, having a good understanding of C can provide a strong foundation for learning C++. C++ is an extension of C and includes all of its features, so familiarity with C can make learning C++ easier.
Yes, it’s possible to learn C and C++ simultaneously. However, since C++ is an extension of C, it’s generally recommended to start with C and then move on to C++.
Absolutely! C and C++ are widely used in various domains, including systems programming, embedded systems, game development, and high-performance computing. Many foundational technologies and frameworks are built using C and C++.
The time required may vary depending on your learning pace and schedule. Aim to spend at least 1-2 hours each day on focused learning and practice to make steady progress.
There are several resources available, including online tutorials, textbooks, coding exercises, and community forums. Choose resources that suit your learning style and provide hands-on practice opportunities.
Yes, you’ll have a solid foundation to start building applications in C and C++. Start with small projects and gradually increase the complexity as you gain more experience and confidence.