Introduction
Flask is a popular Python web framework that allows developers to build web applications quickly and easily. One of the key features of Flask is its support for user authentication and login functionality. In this article, we will cover how to build a Flask login web app, including user registration, login, and authentication. We will also explore the security aspects of building a Flask login web app, including password hashing and session management.shark tank India pitches
Prerequisites
Before we dive into building a Flask login web app, it’s important to have a basic understanding of Python and Flask. Additionally, we will be using Flask-WTF and Flask-Login, two popular Flask extensions that simplify the process of building web forms and implementing user authentication, respectively. Make sure these extensions are installed and running the following commands in your terminal:
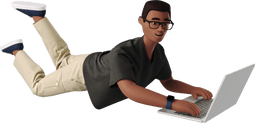
FLask Login Web App
Dependancies
pip install flask-wtf
pip install flask-login
User Registration
The first step in building a Flask login web app is to create a registration form that allows new users to create an account. We will be using Flask-WTF to build our form. Here’s an example registration form:
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, SubmitField
from wtforms.validators import DataRequired, Email, EqualTo
class RegistrationForm(FlaskForm):
username = StringField('Username', validators=[DataRequired()])
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('Password', validators=[DataRequired()])
confirm_password = PasswordField('Confirm Password', validators=[DataRequired(), EqualTo('password')])
submit = SubmitField('Sign Up')
In this form, we have four fields: username, email, password, and confirm_password. Each field has a corresponding validator that ensures the field is not empty or meets specific requirements (such as a valid email format). We also have a SubmitField that will allow the user to submit the form.
Next, we need to create a route that will render this form and handle the user’s form submission. Here’s an example route:
from flask import render_template, redirect, url_for
from your_app import app, db
from your_app.models import User
from your_app.forms import RegistrationForm
@app.route('/register', methods=['GET', 'POST'])
def register():
form = RegistrationForm()
if form.validate_on_submit():
user = User(username=form.username.data, email=form.email.data)
user.set_password(form.password.data)
db.session.add(user)
db.session.commit()
return redirect(url_for('login'))
return render_template('register.html', title='Register', form=form)
In this route, we first create an instance of the RegistrationForm class. We then check with form has been submitted and is valid (i.e., all fields pass their respective validators). If the form is valid, we create a new User object with the data provided by the form and add it to our database. We then redirect the user to the login page. If the form is not valid, we simply render the registration form again.
Login and Authentication
Now that we have a way for users to register for our web app, we need to provide a way for them to log in. For this, we will be using Flask-Login. Here’s an example login form:
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, SubmitField, BooleanField
from wtforms.validators import DataRequired, Email, EqualTo
class LoginForm(FlaskForm):
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('Password', validators=[DataRequired()])
remember = BooleanField('Remember Me')
submit =SubmitField('Log In')
This form has three fields: email, password, and remember. The remember field allows users to stay logged in even after they close their browser. Next, we need to create a route that will handle the user’s login request. Here’s an example route:
from flask import render_template, redirect, url_for, flash, request
from flask_login import login_user, logout_user, current_user
from your_app import app
from your_app.forms import LoginForm
from your_app.models import User
@app.route('/login', methods=['GET', 'POST'])
def login():
if current_user.is_authenticated:
return redirect(url_for('home'))
form = LoginForm()
if form.validate_on_submit():
user = User.query.filter_by(email=form.email.data).first()
if user is None or not user.check_password(form.password.data):
flash('Invalid email or password')
return redirect(url_for('login'))
login_user(user, remember=form.remember.data)
next_page = request.args.get('next')
if not next_page or url_parse(next_page).netloc != '':
next_page = url_for('home')
return redirect(next_page)
return render_template('login.html', title='Log In', form=form)
In this route, we first check if the user is already logged in. If they are, we redirect them to the home page. We then create an instance of the LoginForm class and check if the form has been submitted and is valid. If the form is valid, we query our database for a User object with the email provided by the form. If we find a matching user and their password is correct, we log them in using the login_user() function provided by Flask-Login. We also check if the user was redirected to the login page from another page (i.e., they tried to access a protected page without being logged in). If so, we redirect them back to that page after they log in.
Logout
To allow users to log out of our Flask login web app, we need to create a logout route. Here’s an example route:
from flask_login import logout_user
@app.route('/logout')
def logout():
logout_user()
return redirect(url_for('home'))
In this route, we simply call the logout_user() function provided by Flask-Login and redirect the user to the home page.
Password Hashing
To ensure the security of our users’ passwords, we need to hash them before storing them in our database. We will be using Werkzeug’s security module to generate password hashes. Here’s an example User model that uses password hashing:
from werkzeug.security import generate_password_hash, check_password_hash
from flask_login import UserMixin
from your_app import db, login
class User(UserMixin, db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(64), index=True, unique=True)
email = db.Column(db.String(120), index=True, unique=True)
password_hash = db.Column(db.String(128))
def set_password(self, password):
self.password_hash = generate_password_hash(password)
def check_password(self, password):
return check_password_hash(self.password_hash, password)
@login.user_loader
def load_user(id):
return User.query.get(int(id))
In this model, we use the generate_password_hash() function to hash the user’s password before storing it in the database. We also use the check_password_hash() function to compare the hashed password with a user-provided password during login.
Session Management
To ensure the security of our users’ sessions, we need to configure Flask’s session management
Flask uses the Flask-Session extension to manage sessions. To use Flask-Session, we need to install it using pip:
pip install Flask-Session
Next, we need to configure our app to use Flask-Session. Here’s an example configuration:
from flask import Flask
from flask_session import Session
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key_here'
app.config['SESSION_TYPE'] = 'filesystem'
Session(app)
In this configuration, we set the app’s secret key (used to encrypt session data) and configure the session type to be stored on the file system.
Protecting Routes
Now that we have our login and logout routes set up, we can use Flask-Login’s @login_required decorator to protect certain routes from unauthorized access. Here’s an example route that requires the user to be logged in:
In this route, we use the @login_required decorator to ensure that only logged-in users can access the profile page. If a user is not logged in and tries to access this page, they will be redirected to the login page.
from flask_login import login_required
@app.route('/profile')
@login_required
def profile():
return render_template('profile.html')
Summary
In this article, we covered the basics of building a Flask login web app. I will start by installing and configuring Flask-Login, which provides user authentication and session management. We then created a login form and route, as well as a logout route. We also covered password hashing and protecting routes with the @login_required decorator.
If you want to learn more about building Flask web apps, check out the Flask documentation or my blog at technilesh.com. I hope this article has been helpful, and happy coding!