Hello, It is our 2nd day of learning Javascript, if you come directly here please check out how the javascript is executing in the backend, how the call stack is created how the Execution context is created, and the flow of execution.
in the simple word if you created the function and assigned the variable ( eg. var n=10) inside it. then the variable only scope up to function, then it is called a Local variable.
I think you understand, what will be the global variable? As you think, the variable assigned outside the function becomes the global variable.
Global Variables:
Global variables are declared outside of any function or block of code. They are accessible throughout the entire program, making them global in scope.
// Global variable
var globalVar = "I am global";
function exampleFunction() {
// Accessing the global variable inside a function
console.log(globalVar);
}
exampleFunction(); // Output: "I am global"
Local Variables:
Local variables are declared within a function or a block of code. They are only accessible within the function or block where they are declared.
function exampleFunction() {
// Local variable
var localVar = "I am local";
console.log(localVar);
}
exampleFunction(); // Output: "I am local"
// Attempting to access localVar outside the function will result in an error
// console.log(localVar); // This will cause an error
Example how it working in Backend , Execution stack , Call Stack
var g = 10;
f1();
f2();
console.log(g);
function f1() {
var g = 100;
console.log(g);
}
function f2() {
var g = 19;
console.log(g);
}
//output:
100
19
10
the table show that how the call stack is created and how each function is executed in order.
Execution Context | Local Variables | Console Logs | Call Stack |
---|---|---|---|
Global Context | g = 10 | [Global Context] | |
f1 Context | g = 100 | console.log(100) | [f1, Global Context] |
f2 Context | g = 19 | console.log(19) | [f2, f1, Global Context] |
[f1, Global Context] | |||
console.log(10) | [Global Context] | ||
Program Completion | [] |
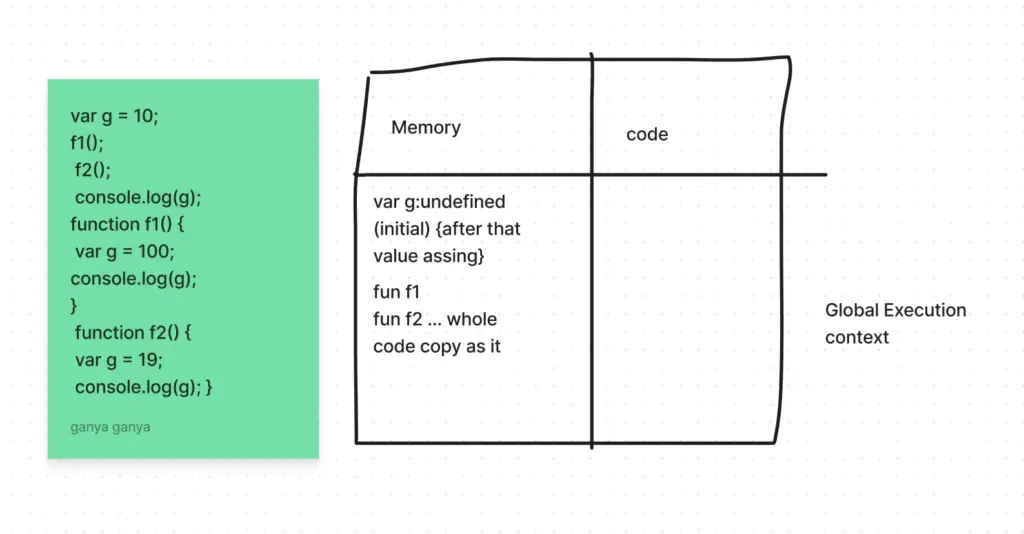
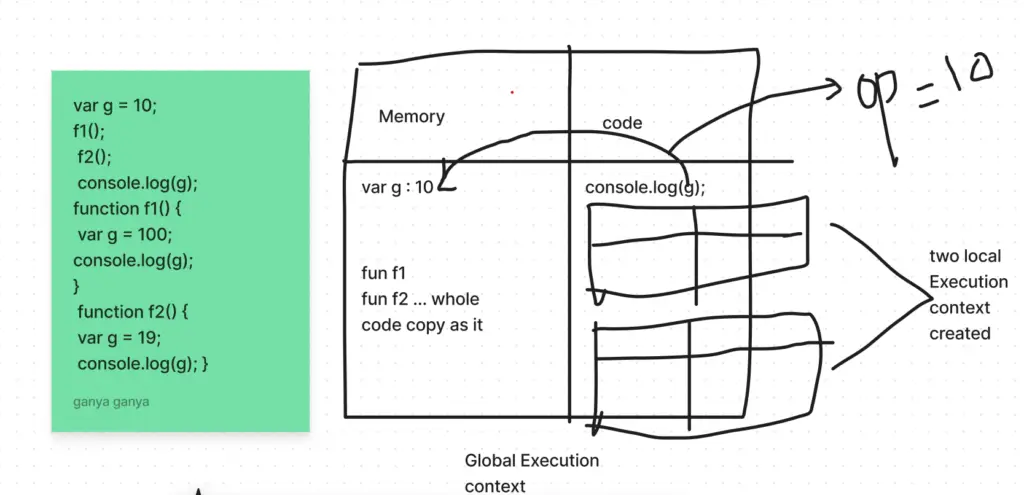
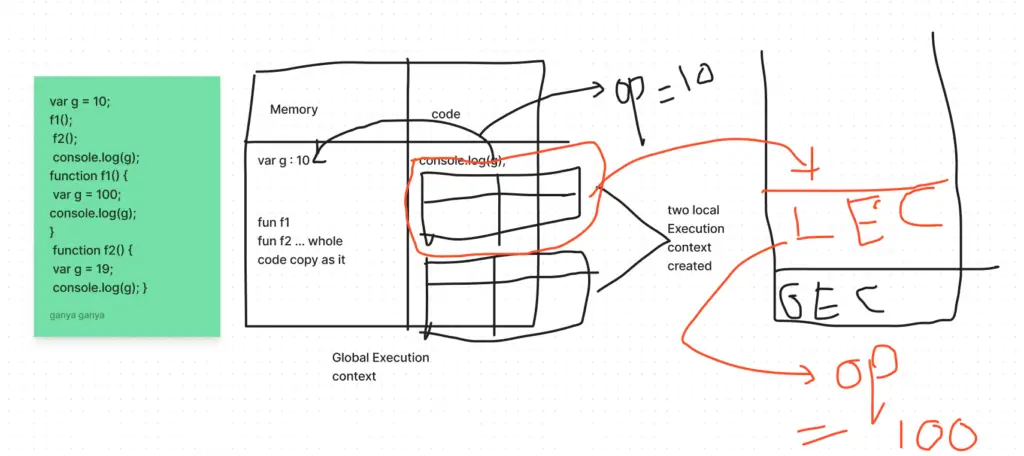
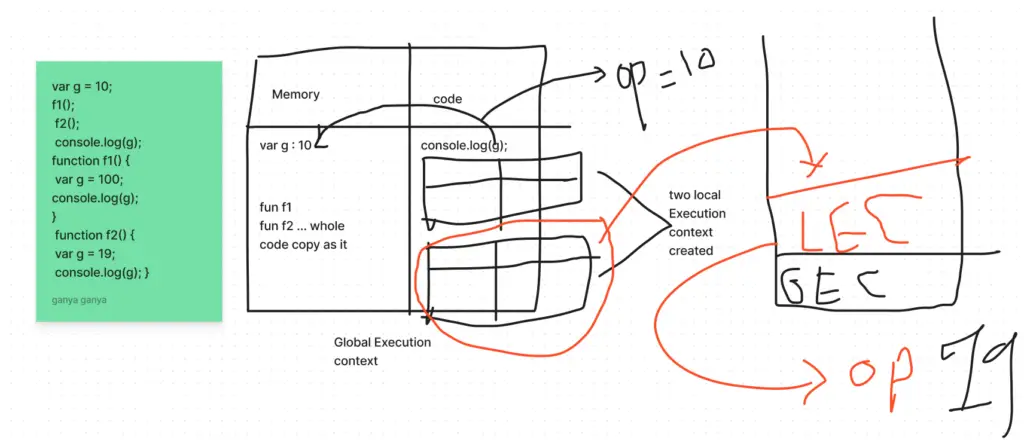