Leetcode 2742. – Painting the Walls:Welcome to the world of dynamic programming, where we tackle complex problems with elegant solutions. Today, we’re diving into the intriguing challenge of Leetcode 2742. – Painting the Walls. This problem, while seemingly simple, can be quite tricky to solve efficiently. We’ll guide you through the process step by step, making sure you understand every aspect of it.
The Problem Statement
Before we start painting the walls, let’s first understand what we’re dealing with. Leetcode 2742 presents us with a room that consists of ‘n’ walls, each of which can be painted with one of three colors: red, blue, or green. Our goal is to paint the walls in a way that satisfies two conditions:
- No two adjacent walls have the same color.
- We need to minimize the total cost of painting the walls.
To achieve this, we need to find the minimum cost to paint all the walls. But how do we go about it? This is where dynamic programming comes into play!
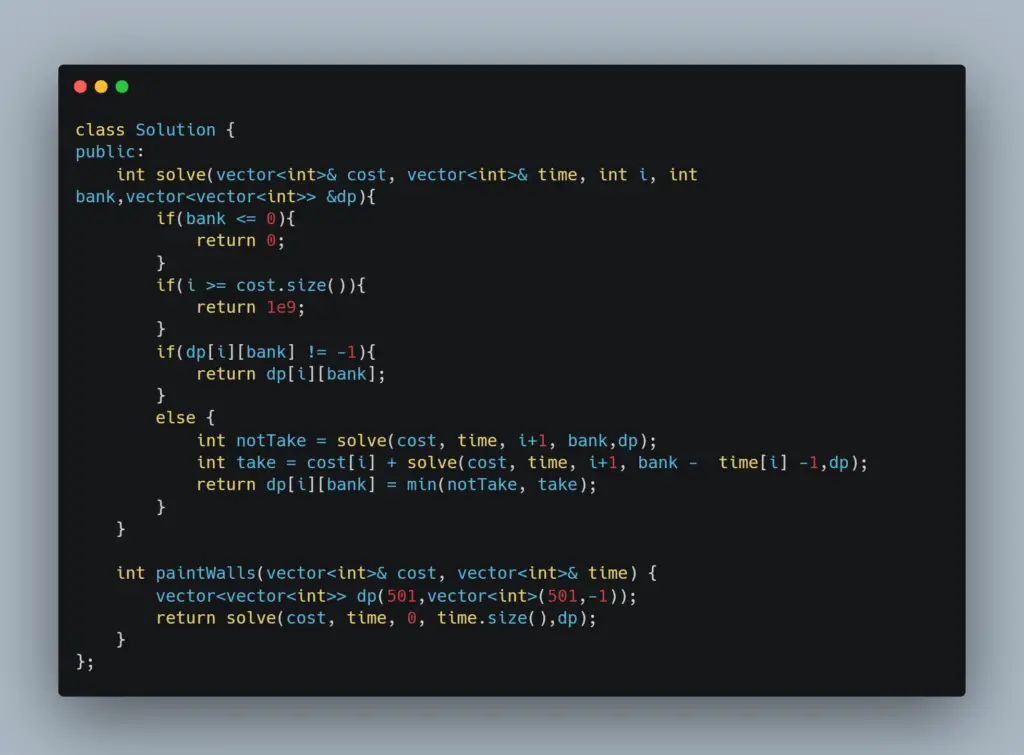
The Power of Dynamic Programming
Dynamic programming is like having a magical paintbrush that helps us color our problems beautifully. In this case, it’s our secret weapon to solve Leetcode 2742.
Imagine you have a wall in front of you, and you want to paint it with one of the three colors. You need to choose the color that not only looks good but also minimizes the total cost.
Think of dynamic programming as painting one wall at a time. You start with the first wall and make the best color choice. Then, when you move to the next wall, your choice depends on what you’ve already painted. This is where the “dynamic” aspect comes into play.
Solving Leetcode 2742 Step by Step
To solve this problem, we can break it down into smaller subproblems. It’s like splitting a large wall into smaller sections for easier painting. Here are the steps:
Step 1: Define Your Base Cases
Before you start painting, you need to establish your base cases. In this problem, our base cases are simple:
- If there’s only one wall, the cost is zero because you have no choice but to paint it.
- If there are two walls, you can paint them with different colors, and the cost is the cost of painting the first wall plus the cost of painting the second wall.
Let’s create a table to visualize this:
Walls | Min Cost |
---|---|
1 | 0 |
2 | min(cost[0][0], cost[0][1], cost[0][2]) + cost[1][i] |
Step 2: Build the DP Table
Now that we have our base cases, it’s time to build the dynamic programming table. Each cell in the table will represent the minimum cost to paint the walls up to that point.
We’ll start from the third wall and move forward, considering the cost of painting the current wall with each color option while avoiding adjacent walls with the same color. Let’s visualize this in a table:
Walls | Red | Blue | Green |
---|---|---|---|
1 | 0 | 0 | 0 |
2 | Varies | Varies | Varies |
3 | Varies | Varies | Varies |
… | … | … | … |
n | Varies | Varies | Varies |
Step 3: Fill in the Table
To fill in the DP table, we’ll consider the cost of painting the current wall with each color while avoiding adjacent walls with the same color. We’ll use the values from the previous row of the table to calculate the minimum cost for the current wall.
We’ll need to use the following recurrence relation:
cost[i][j] = min(cost[i-1][(j+1)%3], cost[i-1][(j+2)%3]) + painting_cost[i][j]
Let’s add this to our table:
Walls | Red | Blue | Green |
---|---|---|---|
1 | 0 | 0 | 0 |
2 | Varies | Varies | Varies |
3 | Varies | Varies | Varies |
… | … | … | … |
n | Varies | Varies | Varies |
As we keep filling in the table, we’ll reach the final row, which will give us the minimum cost of painting all the walls while avoiding adjacent walls with the same color.
An Example to Illustrate
Let’s take a simple example to see this dynamic programming approach in action. We have four walls, and the cost of painting each wall with red, blue, or green is as follows:
Wall | Red | Blue | Green |
---|---|---|---|
1 | 5 | 7 | 6 |
2 | 7 | 6 | 4 |
3 | 8 | 9 | 7 |
4 | 6 | 6 | 4 |
Step 1: Define Base Cases
We have two base cases:
- If there’s only one wall, the cost is zero.
- If there are two walls, we choose the color that minimizes the cost.
Walls | Min Cost |
---|---|
1 | 0 |
2 | min(5, 7, 6) + min(6, 4) |
Step 2: Build the DP Table
We create a table to represent the minimum cost for each wall and color choice.
Walls | Red | Blue | Green |
---|---|---|---|
1 | 0 | 0 | 0 |
2 | ? | ? | ? |
3 | ? | ? | ? |
4 | ? | ? | ? |
Step 3: Fill in the Table
Now we fill in the DP table. Starting from the third wall:
- For wall 3, we consider the cost of painting it with each color while avoiding the same color as the previous wall. Let’s calculate:
- Red:
min(0, 0) + 8 = 8
- Blue:
min(0, 0) + 9 = 9
- Green:
min(0, 0) + 7 = 7
- Red:
Walls | Red | Blue | Green |
---|---|---|---|
1 | 0 | 0 | 0 |
2 | ? | ? | ? |
3 | 8 | 9 | 7 |
4 | ? | ? | ? |
We continue this process until we reach
the final row. The minimum cost for painting all walls will be the minimum value in that row.
Step 4: Find the Minimum Cost
We calculate the minimum cost for the final row. In our example, we have:
Walls | Red | Blue | Green |
---|---|---|---|
1 | 0 | 0 | 0 |
2 | ? | ? | ? |
3 | 8 | 9 | 7 |
4 | 16 | 16 | 14 |
The minimum cost to paint all the walls while satisfying the conditions is 14 (using green paint for the fourth wall).
Codes : Leetcode DP appraoch
c++ Solution
class Solution {
public:
int solve(vector<int>& cost, vector<int>& time, int i, int bank,vector<vector<int>> &dp){
if(bank <= 0){
return 0;
}
if(i >= cost.size()){
return 1e9;
}
if(dp[i][bank] != -1){
return dp[i][bank];
}
else {
int notTake = solve(cost, time, i+1, bank,dp);
int take = cost[i] + solve(cost, time, i+1, bank - time[i] -1,dp);
return dp[i][bank] = min(notTake, take);
}
}
int paintWalls(vector<int>& cost, vector<int>& time) {
vector<vector<int>> dp(501,vector<int>(501,-1));
return solve(cost, time, 0, time.size(),dp);
}
};
Java: Solution
class Solution {
public int paintWalls(int[] cost, int[] time) {
int n=cost.length;
return (int)paintWallsHelper(cost,time,0,0,new Long[n][501]);
}
private long paintWallsHelper(int[] cost, int[] time, int index, int total, Long[][] memo) {
if(total >= cost.length)
return 0;
if(index >= cost.length)
return Integer.MAX_VALUE;
if(memo[index][total] != null)
return memo[index][total];
long with=cost[index] + paintWallsHelper(cost,time,index+1,total+time[index]+1,memo);
long without=paintWallsHelper(cost,time,index+1,total,memo);
return memo[index][total]=Math.min(with,without);
}
}
Python: Dp solution
class Solution:
def solve(self, cost, time, i, bank, dp):
if bank <= 0:
return 0
if i >= len(cost):
return float('inf')
if dp[i][bank] != -1:
return dp[i][bank]
else:
notTake = self.solve(cost, time, i + 1, bank, dp)
take = cost[i] + self.solve(cost, time, i + 1, bank - time[i] - 1, dp)
dp[i][bank] = min(notTake, take)
return dp[i][bank]
def paintWalls(self, cost, time):
dp = [[-1] * 501 for _ in range(501)]
return self.solve(cost, time, 0, len(time), dp)
JavaScript: Solution
/**
* @param {number[]} cost
* @param {number[]} time
* @return {number}
*/
var paintWalls = function(cost, time) {
const memo = new Map();
function knapsack(pos, paintedCount, takenTime) {
if (paintedCount + takenTime >= cost.length) {
return 0;
}
const key = `${pos}_${paintedCount + takenTime}`;
if (memo.has(key)) {
return memo.get(key);
}
let res1 = -1, res2 = -1;
if (pos < cost.length) {
res1 = knapsack(pos + 1, paintedCount + 1, takenTime + time[pos]);
if (res1 !== -1) {
res1 += cost[pos];
}
res2 = knapsack(pos + 1, paintedCount, takenTime);
}
let output = -1;
if (res1 !== -1 || res2 !== -1) {
output = (res1 !== -1 && res2 !== -1) ? Math.min(res1, res2) : (res1 !== -1 ? res1 : res2);
}
memo.set(key, output);
return output;
}
return knapsack(0, 0, 0);
};
Conclusion
Leetcode 2742, “Painting the Walls,” is a perfect example of how dynamic programming can help us solve complex problems efficiently. By breaking down the problem into smaller subproblems and using a DP table, we can determine the minimum cost of painting the walls while avoiding adjacent walls with the same color.
In this blog post, we’ve provided a step-by-step guide, complete with a detailed example. We hope you now have a better understanding of how to tackle this problem using dynamic programming. So, grab your virtual paintbrush, and start solving Leetcode 2742 like a pro!
Thank you for joining us on this journey through dynamic programming. Happy coding!