LeetCode 896. Monotonic Array: Hey there, coding enthusiasts! Welcome back to another exciting coding session. Today’s problem is a treat—literally! We’re going to solve the “Monotonic Array” or “LeetCode .896”
Approach for Leetcode 898: Monotonic Array
- Initialization: We initialize a variable called
check
to 0, representing an unknown state. 1 denotes increasing, and -1 denotes decreasing. - Iterating Through the Array: We traverse the input array, starting from the second element.
- Checking Monotonicity: For each pair of adjacent elements, we compare them to determine if the array is monotonic:
- If the current element is greater than the previous one, we mark it as increasing.
- If the current element is less than the previous one, we mark it as decreasing.
- Validity Check: While checking, if we encounter a conflict (e.g., switching from increasing to decreasing), we return
false
, indicating the array is not monotonic. - Conclusion: If we successfully traverse the entire array without conflicts, we return
true
, indicating that the array is monotonic.
This solution solves the Leetcode problem 898, which checks if an array is monotonic, i.e., either entirely non-increasing or non-decreasing.
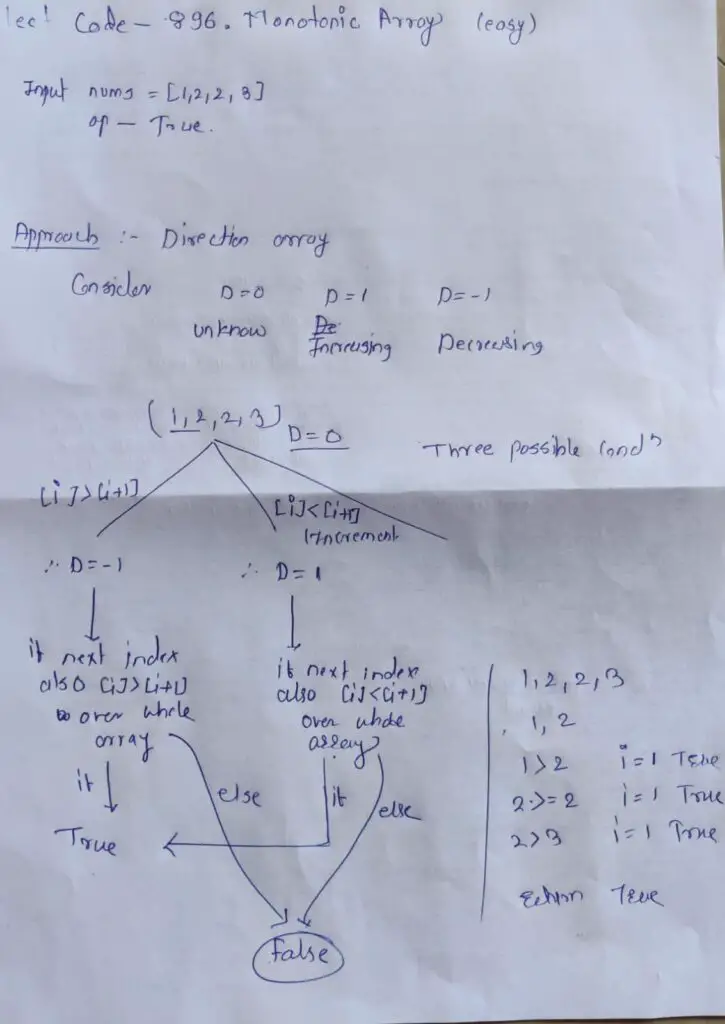
Codes : Approach for Leetcode 898
C++ : Leetcode 898: Monotonic Array
class Solution {
public:
bool isMonotonic(vector<int>& nums) {
if (nums.size() < 2) return true;
int check = 0; // 0 means unknown, 1 means increasing, -1 means decreasing
for (size_t i = 1; i < nums.size(); i++) {
if (nums[i] > nums[i-1]) { // increasing
if (check == 0) check = 1;
else if (check == -1) return false;
}
else if (nums[i] < nums[i-1]) { // decreasing
if (check == 0) check = -1;
else if (check == 1) return false;
}
}
return true;
}
};
Java: Leetcode 898: Monotonic Array
import java.util.*;
public class Solution {
public boolean isMonotonic(int[] nums) {
if (nums.length < 2) return true;
int check = 0; // 0 means unknown, 1 means increasing, -1 means decreasing
for (int i = 1; i < nums.length; i++) {
if (nums[i] > nums[i - 1]) { // increasing
if (check == 0) check = 1;
else if (check == -1) return false;
} else if (nums[i] < nums[i - 1]) { // decreasing
if (check == 0) check = -1;
else if (check == 1) return false;
}
}
return true;
}
}
Python: Leetcode 898: Monotonic Array
class Solution:
def isMonotonic(self, nums: List[int]) -> bool:
if len(nums) < 2:
return True
check = 0 # 0 means unknown, 1 means increasing, -1 means decreasing
for i in range(1, len(nums)):
if nums[i] > nums[i - 1]: # increasing
if check == 0:
check = 1
elif check == -1:
return False
elif nums[i] < nums[i - 1]: # decreasing
if check == 0:
check = -1
elif check == 1:
return False
return True
Leet Code: Rotate Array C++ Python || JavaScript || Java solution
JavaScript: Leetcode 898: Monotonic Array
var isMonotonic = function(nums) {
if (nums.length < 2) return true;
let check = 0; // 0 means unknown, 1 means increasing, -1 means decreasing
for (let i = 1; i < nums.length; i++) {
if (nums[i] > nums[i - 1]) { // increasing
if (check == 0) check = 1;
else if (check == -1) return false;
} else if (nums[i] < nums[i - 1]) { // decreasing
if (check == 0) check = -1;
else if (check == 1) return false;
}
}
return true;
};
Result Analysis Leetcode 898: Monotonic Array
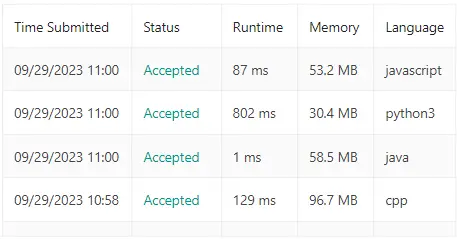