Reverse Linked List :You can solve this problem in C++, Java, Python, or JavaScript using the following algorithm to reverse a singly linked list:
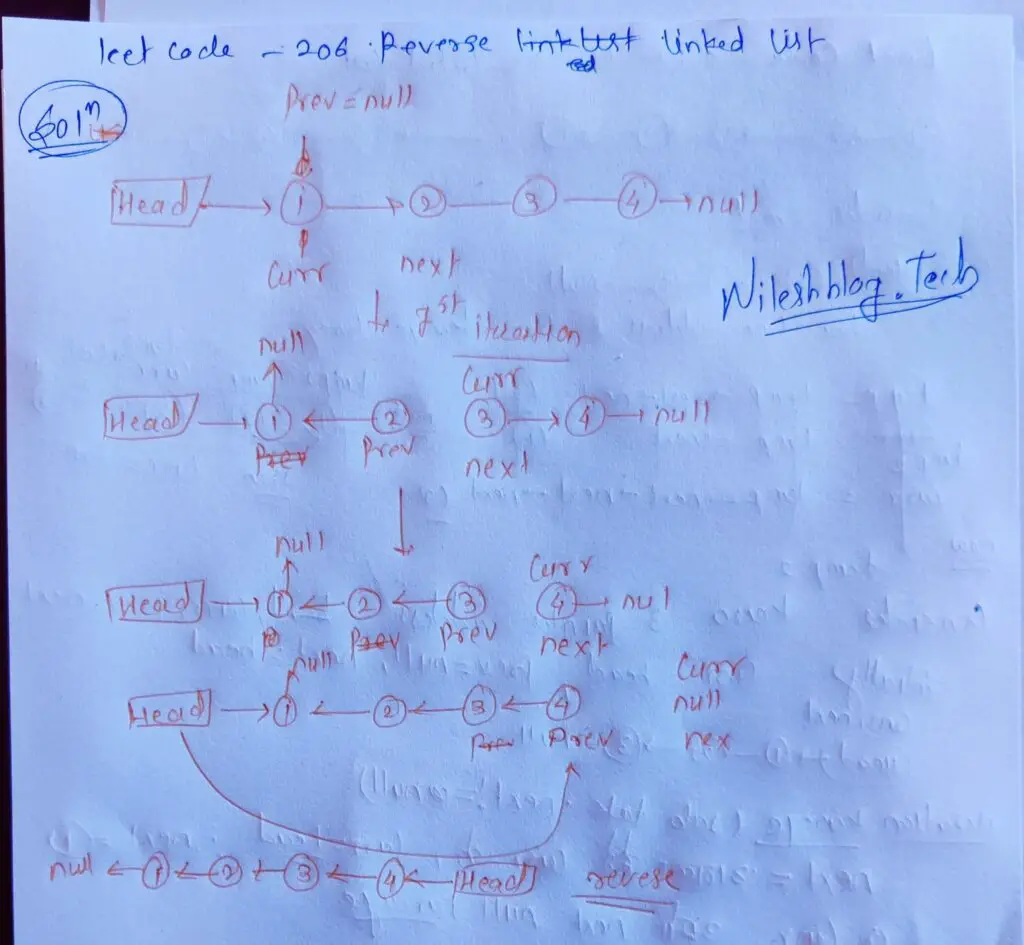
Leet Code : Reverse Nodes in k-Group Java, Python ,C++ JavaScript Solution
Imagine you have a chain of beads, and you want to reverse their order. That’s what this code does to a list of numbers. Let’s break it down:
- We have a list of numbers (like [1, 2, 3, 4, 5]).
- We start with nothing in our hand (that’s like
prev = null
) and look at the first number (that’s likecurrent = 1
). - We take the first number (1) and put it in our other hand. Now our other hand has 1, and the first number (1) is gone.
- We look at the next number (2) and put it in our other hand. Now our other hand has 2, and the list looks like [1, 3, 4, 5].
- We keep doing this until there are no more numbers in the list.
- Finally, our other hand has all the numbers in reverse order (like [5, 4, 3, 2, 1]).
So, the code takes a list of numbers, starts with an empty hand (prev
), and goes through the list, putting the numbers in reverse order in the other hand. When it’s done, it gives you the reversed list.
That’s it! The code helps us reverse a list of numbers just like turning a chain of beads around.
Here’s the code in C++, Java, Python, and JavaScript:
Leet Code :Linked List Cycle II Java || Python || C++ solution
C++:Leet code 206
class ListNode {
public:
ListNode* reverseList(ListNode* head) {
ListNode* prev = nullptr;
ListNode* current = head;
while (current != nullptr) {
ListNode* next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev; // prev will be the new head of the reversed list
}
Leet Code : Intersection of Two Linked ListsLeet Code – Java | Python | C++ | Dart | Easy Solution
Java: Leet code 206
class ListNode {
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode current = head;
while (current != null) {
ListNode next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev; // prev will be the new head of the reversed list
}
Python: Reverse Linked List
class ListNode:
def reverseList(head):
prev = None
current = head
while current is not None:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev # prev will be the new head of the reversed list
JavaScript: Reverse Linked List
var reverseList = (head) => {
let prev = null;
let current = head;
while (current !== null) {
const nextNode = current.next;
current.next = prev;
prev = current;
current = nextNode;
}
return prev; // prev will be the new head of the reversed list
};
Please share with Your college friends and follow our site 100 days Leet code challenge.