In the dynamic realm of JavaScript, promises stand as a pivotal concept, transforming the landscape of asynchronous programming. This comprehensive guide will navigate you through the intricacies of promises, covering everything from their definition and creation to the call stack and beyond.
Understanding Promises in JavaScript
Defining Promises
At its core, a promise is an object representing the eventual completion or failure of an asynchronous operation. It is a placeholder for the result of that operation, whether it’s resolving with a value or rejecting with a reason.
Creation of Promises in Javascript
Creating promises involves the use of the Promise
constructor. We’ll delve into the syntax and explore various examples to solidify your understanding of how promises are initialized.
Asynchronous Programming with Promises
Basics of Asynchronous Programming
Before diving into promises, it’s essential to understand the fundamentals of asynchronous programming. We’ll explore callback functions, the event loop, and how JavaScript manages asynchronous tasks.
Resolve and Reject Methods
The resolve
and reject
methods play a pivotal role in the promise lifecycle. Learn how these methods determine whether a promise should be fulfilled with a value or rejected with a reason.
Chaining Promises
Chaining promises is a powerful technique for handling multiple asynchronous tasks. We’ll walk through examples, illustrating how to create a seamless flow of operations by chaining promises effectively.
Error Handling with Promises
Error handling is a critical aspect of writing robust and reliable code. Discover how promises simplify error handling and explore best practices for gracefully managing errors in asynchronous operations.
States of a Promise: Pending, Fulfilled, and Rejected
Pending State
A promise starts in the pending state, indicating that the asynchronous operation is ongoing. Explore scenarios where promises remain in the pending state.
Fulfilled State
When a promise successfully completes its operation, it enters the fulfilled state. Learn how to handle and retrieve values from fulfilled promises.
Rejected State
In case of an error or unsuccessful operation, a promise enters the rejected state. Understand how to gracefully handle rejections and retrieve the reason for failure.
The Call Stack and Promises
Understanding the call stack is crucial for grasping how promises function. We’ll unravel the behind-the-scenes work, exploring the event loop and how promises interact with the call stack.
Pros and Cons of Using Promises
Pros
- Asynchronous Simplicity: Promises streamline asynchronous code, making it more readable and maintainable.
- Error Handling: Promises provide a structured way to handle errors, enhancing code reliability.
- Chaining: Learn the art of chaining promises, allowing for elegant sequencing of asynchronous tasks.
Cons
- Complexity for Beginners: The concept of promises can be challenging for beginners, requiring a shift in mindset.
- Promise Hell: Improper chaining may lead to “promise hell,” making code hard to follow.
Behind-the-Scenes Work: Event Loop and States
Event Loop in JavaScript
Understanding the event loop is crucial for mastering promises. We’ll dissect the event loop’s role in managing asynchronous operations and maintaining the smooth execution of your code.
Pending, Fulfilled, and Rejected States
Explore the three states a promise can exist in: pending, fulfilled, and rejected. Learn how these states impact the flow of your asynchronous operations.
Tips for Effective Promise Usage
- Chaining Promises Effectively: Master the art of chaining promises to create a seamless flow of asynchronous tasks.
- Error Handling Best Practices: Implement robust error handling strategies to fortify the reliability of your code.
- Utilizing Promise.All: Discover the power of
Promise.all
for handling multiple promises concurrently.
Real Word Example of Promise in Javascript
Code Explanation
- Array Initialization:
const transactions = ["debit", "credit"];
This line initializes an array named transactions
with two elements: “debit” and “credit.” In a banking context, these transactions could represent different types of financial operations.
makeTransaction
Function:
function makeTransaction(transactions){
return new Promise(function(resolve, reject){
if(!transactions){
const errorMessage = "Error: No transactions found";
reject(errorMessage);
}
else{
const transactionId = 456;
resolve(transactionId);
}
});
}
makeTransaction
is a function that takes an array of transactions as a parameter.- It returns a Promise that either resolves with a transaction ID (if transactions are present) or rejects with an error message.
- Promise Chaining (
.then
and.catch
):
makeTransaction(transactions)
.then(function(transactionId){
console.log(transactionId);
return transactionId;
}).catch(function(error){
console.log(error);
})
- The
makeTransaction
function is invoked with thetransactions
array. - The first
.then
block logs the transaction ID if the promise is resolved. - The
.catch
block logs an error message if the promise is rejected.
initiatePayment
Function:
function initiatePayment(transactionId){
const transactionIdentifier = transactionId;
return new Promise(function(resolve, reject){
if(transactionIdentifier){
const paymentMessage = "Payment successful";
resolve(paymentMessage);
}
const errorMessage = "Error: Payment failed";
reject(errorMessage);
});
}
initiatePayment
takes atransactionId
as a parameter and returns a Promise.- It simulates a payment process, resolving with a success message if the transaction ID is present and rejecting with an error message otherwise.
JavaScript : Execution Context ,Call Stack ,JavaScript Execution ?(Important to Understand)
- Promise Chaining (
.then
and.catch
):
.then(function(transactionId){
initiatePayment(transactionId).then(result => console.log(result));
return initiatePayment(transactionId);
}).catch(function(error){
console.log(error);
})
- The
initiatePayment
function is chained after the firstmakeTransaction
promise. - It logs the payment result if the promise is resolved and logs an error message if the promise is rejected.
updateBalance
Function:
function updateBalance(paymentStatus){
const paymentResult = paymentStatus;
return new Promise(function(resolve, reject){
if(paymentResult){
const balanceUpdateMessage = "Balance updated successfully";
resolve(balanceUpdateMessage);
}
else{
const errorMessage = "Error: Balance not updated";
reject(errorMessage);
}
});
}
updateBalance
takes apaymentStatus
as a parameter and returns a Promise.- It simulates updating the balance, resolving with a success message if the payment status is present and rejecting with an error message otherwise.
- Promise Chaining (
.then
and.catch
):
.then(function(paymentResult){
return updateBalance(paymentResult)
}).catch(function(error){
console.log(error);
})
- The
updateBalance
function is chained after the secondinitiatePayment
promise. - It logs an error message if the promise is rejected.
- Final
.then
Block:
.then(result => console.log(result))
- This block logs the final result (either success message or error message) of the last promise in the chain.
In summary, the code simulates a series of asynchronous operations in a banking system, where a transaction is made, a payment is initiated, and the balance is updated. Each step is represented by a Promise, and the code uses .then
and .catch
to handle the results of these asynchronous operations.
Full Code of Banking Transaction system using Promise in Javascrip
const transactions = ["debit", "credit"];
makeTransaction(transactions)
.then(function(transactionId){
console.log(transactionId);
return transactionId;
}).catch(function(error){
console.log(error);
})
.then(function(transactionId){
initiatePayment(transactionId).then(result => console.log(result));
return initiatePayment(transactionId);
}).catch(function(error){
console.log(error);
})
.then(function(paymentResult){
return updateBalance(paymentResult)
}).catch(function(error){
console.log(error);
})
.then(result => console.log(result))
function makeTransaction(transactions){
return new Promise(function(resolve, reject){
if(!transactions){
const errorMessage = "Error: No transactions found";
reject(errorMessage);
}
else{
const transactionId = 456;
resolve(transactionId);
}
});
}
function initiatePayment(transactionId){
const transactionIdentifier = transactionId;
return new Promise(function(resolve, reject){
if(transactionIdentifier){
const paymentMessage = "Payment successful";
resolve(paymentMessage);
}
const errorMessage = "Error: Payment failed";
reject(errorMessage);
});
}
function updateBalance(paymentStatus){
const paymentResult = paymentStatus;
return new Promise(function(resolve, reject){
if(paymentResult){
const balanceUpdateMessage = "Balance updated successfully";
resolve(balanceUpdateMessage);
}
else{
const errorMessage = "Error: Balance not updated";
reject(errorMessage);
}
});
}
OutPut of Above Code :
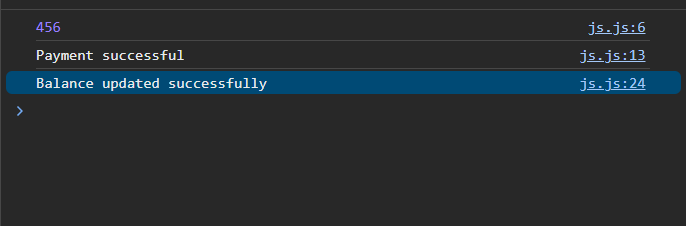
Trusted Reference Sources
- MDN Web Docs – Promises: Link
Your support will help me continue to bring new content. Love Coding! 🌐❤️
Conclusion
As we conclude our journey through the promises landscape, it’s evident that mastering this asynchronous powerhouse is essential for any JavaScript developer. Embrace the pros, navigate the cons, and harness the full potential of promises to elevate your code.