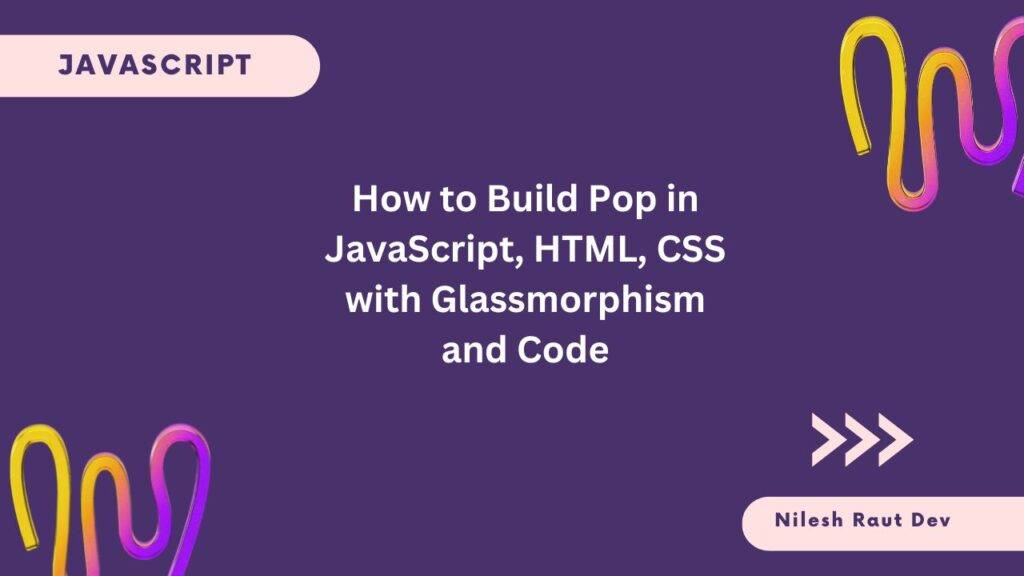
Hey there, fellow developers! Are you looking to add some pizzazz to your website? Want to create eye-catching, modern pop-up elements that will captivate your users? Well, you’re in luck because in this article, we’re going to dive into the exciting world of creating pops using JavaScript, HTML, and CSS, and give them a trendy glassmorphism effect! So, buckle up and get ready to take your web design skills to the next level!.same way You can Build a Instagram Using Html ,JS,CSS .You can Learn the Javascript in 1 Hour with this article : 1 Hour Javascript
1. Introduction to Glassmorphism
What is Glassmorphism?
Glassmorphism is a modern design trend that creates a glass-like, translucent effect for UI elements, giving them a frosted glass appearance. This effect adds depth and dimension to the design, making it visually appealing and stylish. It’s a great way to make your website stand out and leave a lasting impression on your visitors.
Why Glassmorphism is Trending in Web Design
In recent years, glassmorphism has gained immense popularity due to its ability to blend beautifully with various website themes and styles. It brings a touch of elegance and sophistication to the user interface without overpowering the overall design. With glassmorphism, you can create eye-catching pops that look sleek and modern, ensuring your website remains ahead of the curve in terms of aesthetics.
2. Getting Started
Before we jump into the coding magic, let’s set up our project structure and link the necessary JavaScript, HTML, and CSS files. Creating a well-organized project will make it easier to manage and maintain your code as it grows.
Setting Up the Project Structure
Start by creating a new project folder. Inside this folder, create separate sub-folders for your CSS, JavaScript, and any other assets you might use, like images or fonts. Keeping things organized from the start will save you from headaches down the road.
Read ALso : How to Reach to Shegaon
Linking JavaScript, HTML, and CSS Files
Now that our project structure is in place, create a new HTML file in the project’s root folder. This will be the main file where we’ll build our pop. Link the CSS and JavaScript files by adding the following code inside the <head>
section of your HTML file:
htmlCopy code<!-- Linking CSS -->
<link rel="stylesheet" href="css/styles.css">
<!-- Linking JavaScript -->
<script src="js/main.js"></script>
With this setup, we’re ready to start building our glassmorphism pop!
3. Creating the HTML Structure
The foundation of any great pop is its HTML structure. Let’s set up the necessary HTML elements to create our pop.
Setting Up the Pop Container
Our pop will be contained within a <div>
element with a unique identifier. This will allow us to easily target and style the pop using CSS and JavaScript.
htmlCopy code<div class="pop" id="myPop">
<!-- Pop content will go here -->
</div>
Adding Content to the Pop
Now, let’s add some content to our pop. This can include text, images, buttons, or any other elements you want to display inside the pop.
htmlCopy code<div class="pop" id="myPop">
<h2>Welcome to Our Website!</h2>
<p>Thank you for visiting us. We're excited to have you here!</p>
<button>Get Started</button>
</div>
Styling the Pop Container
Before we proceed with the glassmorphism effect, let’s style the pop container using CSS.
4. Styling with CSS
Now that we have the HTML structure in place, it’s time to add the stunning glassmorphism effect to our pop using CSS.
Implementing the Glassmorphism Effect
To achieve the glass-like appearance, we’ll use a combination of background-color
and box-shadow
properties. The key is to set a semi-transparent background color and apply a subtle box-shadow that creates the frosted glass illusion.
cssCopy code/* CSS for the pop container */
.pop {
background-color: rgba(255, 255, 255, 0.85); /* Semi-transparent white background */
box-shadow: 0 8px 32px rgba(31, 38, 135, 0.37); /* Subtle box-shadow */
border-radius: 12px; /* Rounded corners for a softer look */
padding: 20px; /* Add padding to create some space between content and container */
}
Output :
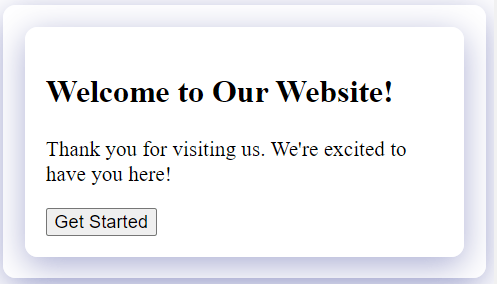
Customizing the Glassmorphism Colors
You can customize the glassmorphism colors to match your website’s theme. Play around with the RGBA values to create the perfect blend for your pop.
cssCopy code/* Customizing glassmorphism colors */
.pop {
background-color: rgba(255, 218, 185, 0.9); /* Semi-transparent peach background */
box-shadow: 0 8px 32px rgba(255, 99, 71, 0.2); /* Subtle box-shadow with a hint of red */
}
Output:
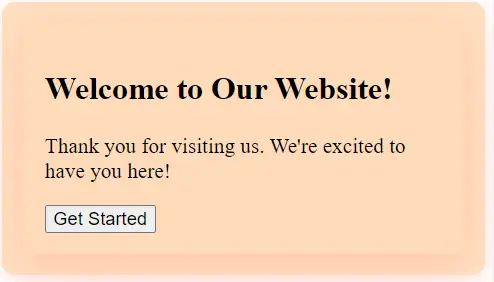
Fine-Tuning the Pop’s Design
Now that our glassmorphism effect is in place, you can fine-tune the design to your liking. Add padding, adjust font sizes, and play with different colors to make the pop truly unique.
cssCopy code/* Fine-tuning the pop's design */
.pop {
padding: 30px; /* Increased padding for more space between content and container */
font-size: 18px; /* Larger font size for better readability */
color: #333; /* Darker text color for contrast */
}
Output:
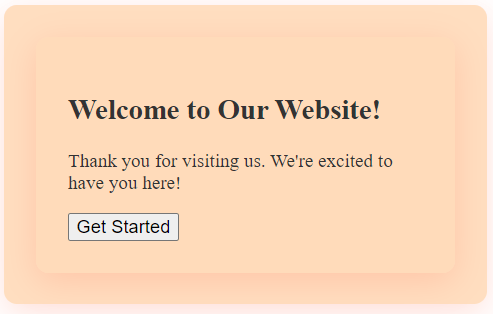
5. Interactivity with JavaScript
Our pop looks fantastic, but it’s currently static. To make it interactive, we’ll use JavaScript to show and hide the pop based on user actions.
Showing and Hiding the Pop
We’ll start by defining functions to show and hide the pop. We’ll use the getElementById
method to access the pop container and toggle its visibility.
javascriptCopy code// JavaScript for showing and hiding the pop
function showPop() {
document.getElementById("myPop").style.display = "block";
}
function hidePop() {
document.getElementById("myPop").style.display = "none";
}
Output:
Triggering the Pop on User Actions
Next, we’ll trigger the pop to show when the user clicks on a button, link, or any other element of your choice.
javascriptCopy code// JavaScript for triggering the pop
const triggerElement = document.getElementById("triggerButton"); // Replace "triggerButton" with your element's ID
triggerElement.addEventListener("click", showPop);
Adding Smooth Animations
To make our pop look even more appealing, let’s add some smooth animations using CSS transitions.
cssCopy code/* CSS for adding smooth animations */
.pop {
transition: all 0.3s ease; /* Smooth transition for pop */
}
.pop.hidden {
opacity: 0; /* Hide the pop when not displayed */
pointer-events: none; /* Disable pointer events when hidden */
}
With these animations in place, our pop will gracefully fade in and out when shown or hidden.
6. Making it Responsive
In today’s mobile-first world, it’s crucial to ensure our pops look great on all devices. Let’s make our pop responsive and adapt its design for different screen sizes.
Ensuring Mobile-Friendly Pops
To ensure our pop adapts well to smaller screens, we’ll use media queries to adjust its size and font for mobile devices.
cssCopy code/* CSS for mobile-friendly pops */
@media screen and (max-width: 768px) {
.pop {
font-size: 16px; /* Smaller font size for better readability on mobile */
padding: 15px; /* Reduced padding for more compact display */
}
}
Adapting the Design for Different Screen Sizes
In addition to font and padding adjustments, you can modify other CSS properties to fine-tune the pop’s design for specific screen sizes.
cssCopy code/* CSS for larger screens */
@media screen and (min-width: 1200px) {
.pop {
width: 400px; /* Wider pop for larger screens */
}
}
7. Testing and Debugging
Before unleashing our pop on the world, it’s crucial to test and debug thoroughly to ensure everything runs smoothly across different browsers and devices.
Cross-Browser Compatibility
Test your pop on various browsers such as Google Chrome, Mozilla Firefox, Microsoft Edge, and Safari. Ensure that the glassmorphism effect and interactive features work as expected.
Identifying and Fixing Common Issues
During testing, you might encounter issues like unexpected behavior, misalignments, or browser-specific quirks. Inspect the browser console for any error messages and address them promptly.
8. Optimizing Performance
Optimizing performance is essential for creating a snappy and delightful user experience. Let’s explore ways to make our pop load faster and efficiently.
Minimizing CSS and JavaScript
Reduce the size of your CSS and JavaScript files by removing unnecessary code and comments. Additionally, consider using CSS and JavaScript minification tools to compress the files further.
Lazy Loading Resources
If your pop contains images or other resources, use lazy loading to load them only when they are about to be displayed. This can significantly improve page loading times, especially on content-heavy pages.
9. Going Beyond the Basics
Now that you’ve mastered the art of creating glassmorphism pops, why stop there? Let’s explore some advanced techniques to take your pops to the next level.
Adding Additional Effects and Transitions
Get creative and experiment with various CSS effects and transitions to enhance the visual appeal of your pops. Explore gradients, animations, and other modern design trends.
Creating Multiple Pops on the Same Page
Why settle for just one pop when you can have multiple! Utilize JavaScript to create and manage multiple pops on the same page, each with its own unique content and design.
10. Accessibility Matters
As responsible developers, it’s crucial to ensure that our pops are accessible to all users, including those with disabilities. Let’s make our pops more inclusive with some accessibility best practices.
Implementing ARIA Attributes
Use ARIA (Accessible Rich Internet Applications) attributes to provide additional information to assistive technologies and make your pops more understandable for screen readers.
htmlCopy code<div class="pop" id="myPop" role="dialog" aria-modal="true">
<!-- Pop content will go here -->
</div>
Keyboard Navigation and Focus Management
Ensure that users can navigate and interact with your pops using only the keyboard. Use the tabindex
attribute to control the order in which elements receive focus.
11. Security Considerations
Security is paramount in web development, even when it comes to creating pops. Let’s explore some essential security considerations to keep our pops and websites safe from common vulnerabilities.
Preventing Common Vulnerabilities
Use secure coding practices and sanitize user input to prevent common security issues like cross-site scripting (XSS) attacks.
Sanitizing User Input
If your pop allows user input, be sure to validate and sanitize it properly to prevent malicious code from being injected into your website.
12. Real-World Examples
Now that you’ve mastered creating glassmorphism pops, let’s get inspired by some real-world examples. Explore popular websites and see how they use pops to engage and delight their users.
13. Troubleshooting and FAQs
While building and implementing pops, you might encounter some challenges. Here are some common troubleshooting tips and frequently asked questions to help you along the way.
How do I center the pop on the screen?
To center the pop both horizontally and vertically, use the following CSS:
cssCopy code.pop {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
Can I use glassmorphism in older browsers?
The glassmorphism effect relies on CSS properties that might not be fully supported in older browsers like Internet Explorer. For better compatibility, consider using fallback styles or progressive enhancement techniques.
Why is my pop not showing up?
Check if you’ve correctly linked your JavaScript file, added the necessary event listeners, and if there are any errors in the browser console.
How can I change the pop’s size?
Adjust the width
and height
properties in the CSS to change the pop’s dimensions. Remember to consider responsiveness for different screen sizes.
What’s the best way to add pop-up analytics?
You can integrate analytics tools like Google Analytics or custom tracking events to monitor user interactions with your pops. Track button clicks, pop views, and other relevant data to gather insights and make data-driven decisions.
Conclusion
Congratulations, you’ve mastered the art of creating stylish and interactive pops with a touch of glassmorphism using JavaScript, HTML, and CSS! By following this guide and experimenting with different designs, you can take your web development skills to new heights and create mesmerizing user experiences. Remember to keep the user in mind, stay updated with the latest trends, and always strive for accessibility and security. So go ahead, get creative, and let your pops shine on the web! Happy coding!