Image Overlap Leetcode:Initially, this problem provides an engaging opportunity for solving as one would eventually discover its enjoyment factor. Moreover, it holds practical significance in real-world scenarios as well.
For instance, by determining the maximal overlapping region between two images and subsequently clipping them accordingly, one can enhance their focus and reduce their size effectively. In this article’s context, we will delve into three distinct approaches:
Also Read : Rain Water Problem in Leetcode
Firstly relying on intuitive enumeration of all feasible overlapping regions; Secondly employing knowledge from linear algebra or geometry for a more efficient solution; Lastly utilizing convolution concepts akin to Convolutional Neural Networks (CNNs), which serve as fundamental operations for contemporary image recognition models.
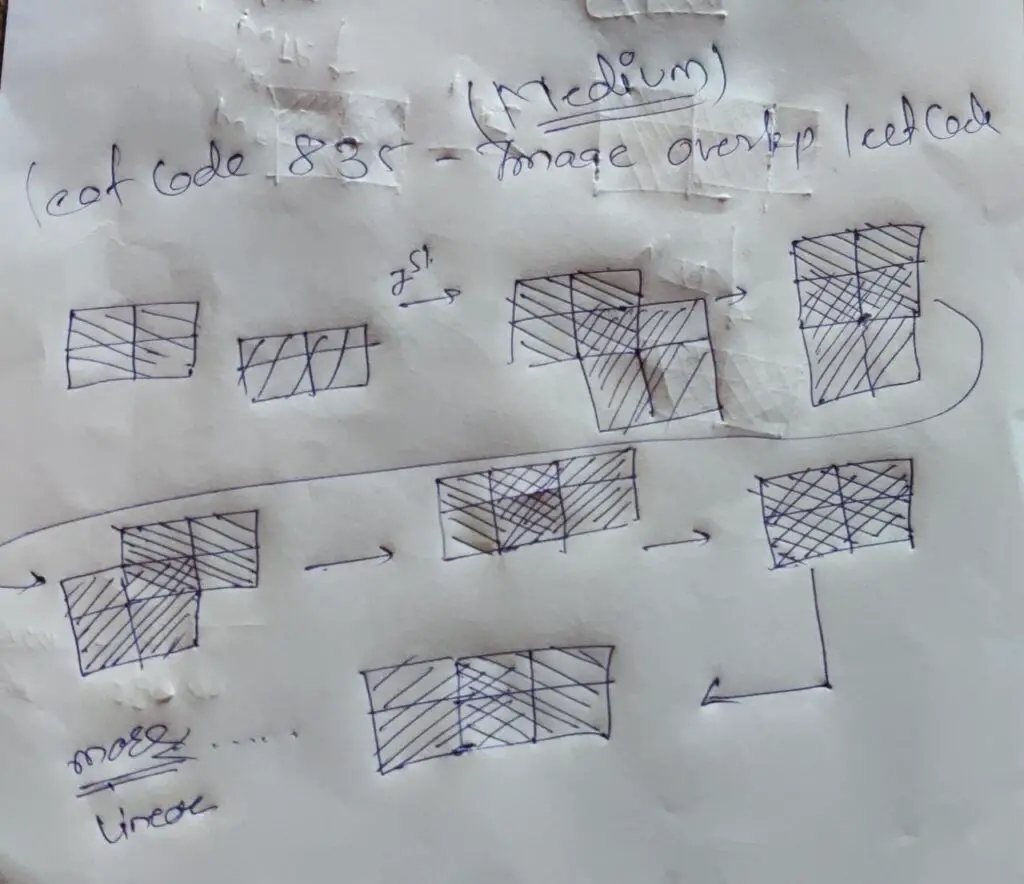
Approach 1: Shift and Count Intuition
As stated in the problem description, calculating the number of ones in the overlapping region necessitates shifting one of the images. Once shifted, it becomes instinctive to perform a simple counting procedure.
Hence, a straightforward idea would involve generating all possible overlapping regions by shifting the image matrix and subsequently counting within each region.
These shifts can be executed in four directions: left, right, up, and down. We can represent these shifts using a two-axis coordinate system where the X-axis denotes left/right shifts and the Y-axis signifies up/down shifts.
Read : Types of Complexities
To implement the solution, we can follow these steps:
- 1: Define the shift function and count function. The shift function shifts the matrix M with reference to matrix R using the given shifting coordinates (x shift, y shift). Then, the count function counts the number of overlapping ones in the overlapping zone.
- 2: Organize the algorithm as a loop. Iterate over all possible combinations of shifting coordinates (x shift, y shift), where both x shift and y shift range from 0 to N-1, where N is the width of the matrix.
- 3: At each iteration, invoke the shift and count functions twice. First, use matrix B as a reference to shift and count the overlapping zone. Then, do vice versa – use matrix R as a reference to perform shifting and counting.
Here’s the provided Java code translated into C++, Java, Python, and JavaScript. The code is essentially the same in all languages, with slight syntax differences.
C++: Image Overlap Shift and Count Intuition
#include <vector>
using namespace std;
class Solution {
public:
int shiftAndCount(int xShift, int yShift, vector<vector<int>>& M, vector<vector<int>>& R) {
int leftShiftCount = 0, rightShiftCount = 0;
int rRow = 0;
for (int mRow = yShift; mRow < M.size(); ++mRow) {
int rCol = 0;
for (int mCol = xShift; mCol < M.size(); ++mCol) {
if (M[mRow][mCol] == 1 && M[mRow][mCol] == R[rRow][rCol])
leftShiftCount += 1;
if (M[mRow][rCol] == 1 && M[mRow][rCol] == R[rRow][mCol])
rightShiftCount += 1;
rCol += 1;
}
rRow += 1;
}
return max(leftShiftCount, rightShiftCount);
}
int largestOverlap(vector<vector<int>>& A, vector<vector<int>>& B) {
int maxOverlaps = 0;
for (int yShift = 0; yShift < A.size(); ++yShift) {
for (int xShift = 0; xShift < A.size(); ++xShift) {
maxOverlaps = max(maxOverlaps, shiftAndCount(xShift, yShift, A, B));
maxOverlaps = max(maxOverlaps, shiftAndCount(xShift, yShift, B, A));
}
}
return maxOverlaps;
}
};
Java: Image Overlap Shift and Count Intuition
import java.util.Arrays;
class Solution {
protected int shiftAndCount(int xShift, int yShift, int[][] M, int[][] R) {
int leftShiftCount = 0, rightShiftCount = 0;
int rRow = 0;
for (int mRow = yShift; mRow < M.length; ++mRow) {
int rCol = 0;
for (int mCol = xShift; mCol < M.length; ++mCol) {
if (M[mRow][mCol] == 1 && M[mRow][mCol] == R[rRow][rCol])
leftShiftCount += 1;
if (M[mRow][rCol] == 1 && M[mRow][rCol] == R[rRow][mCol])
rightShiftCount += 1;
rCol += 1;
}
rRow += 1;
}
return Math.max(leftShiftCount, rightShiftCount);
}
public int largestOverlap(int[][] A, int[][] B) {
int maxOverlaps = 0;
for (int yShift = 0; yShift < A.length; ++yShift) {
for (int xShift = 0; xShift < A.length; ++xShift) {
maxOverlaps = Math.max(maxOverlaps, shiftAndCount(xShift, yShift, A, B));
maxOverlaps = Math.max(maxOverlaps, shiftAndCount(xShift, yShift, B, A));
}
}
return maxOverlaps;
}
}
Python: Image Overlap Shift and Count Intuition
class Solution:
def shiftAndCount(self, xShift, yShift, M, R):
leftShiftCount = 0
rightShiftCount = 0
rRow = 0
for mRow in range(yShift, len(M)):
rCol = 0
for mCol in range(xShift, len(M)):
if M[mRow][mCol] == 1 and M[mRow][mCol] == R[rRow][rCol]:
leftShiftCount += 1
if M[mRow][rCol] == 1 and M[mRow][rCol] == R[rRow][mCol]:
rightShiftCount += 1
rCol += 1
rRow += 1
return max(leftShiftCount, rightShiftCount)
def largestOverlap(self, A, B):
maxOverlaps = 0
for yShift in range(len(A)):
for xShift in range(len(A)):
maxOverlaps = max(maxOverlaps, self.shiftAndCount(xShift, yShift, A, B))
maxOverlaps = max(maxOverlaps, self.shiftAndCount(xShift, yShift, B, A))
return maxOverlaps
JavaScript: Image Overlap Shift and Count Intuition
class Solution {
shiftAndCount(xShift, yShift, M, R) {
let leftShiftCount = 0;
let rightShiftCount = 0;
let rRow = 0;
for (let mRow = yShift; mRow < M.length; ++mRow) {
let rCol = 0;
for (let mCol = xShift; mCol < M.length; ++mCol) {
if (M[mRow][mCol] === 1 && M[mRow][mCol] === R[rRow][rCol]) {
leftShiftCount += 1;
}
if (M[mRow][rCol] === 1 && M[mRow][rCol] === R[rRow][mCol]) {
rightShiftCount += 1;
}
rCol += 1;
}
rRow += 1;
}
return Math.max(leftShiftCount, rightShiftCount);
}
largestOverlap(A, B) {
let maxOverlaps = 0;
for (let yShift = 0; yShift < A.length; ++yShift) {
for (let xShift = 0; xShift < A.length; ++xShift) {
maxOverlaps = Math.max(maxOverlaps, this.shiftAndCount(xShift, yShift, A, B));
maxOverlaps = Math.max(maxOverlaps, this.shiftAndCount(xShift, yShift, B, A));
}
}
return maxOverlaps;
}
}
Alternative Approach: Transforming Image Overlap
One limitation of the aforementioned algorithm is that it repeatedly scans through zero-filled zones, even though these zones are not relevant to our objective.
Despite shifting them, these cells with zeros will not contribute to the final solutions. To address this issue, we can explore a different approach that focuses solely on cells containing ones.
To achieve this, we employ a linear transformation technique to align and compare the relevant cells in both matrices.
Initially, we establish a two-dimensional coordinate system that assigns a unique coordinate to each cell in the matrix. For instance, a cell can be indexed as (x,y).
Building upon this insight, we utilize a transformation vector Vab as a key element to group all non-zero cell alignments between the two matrices.
Each group represents an overlapping zone between the images. The size of each overlapping zone corresponds to the size of its respective group.
Algorithm:
The implementation of this alternative approach involves two main steps.
Firstly, we filter out all non-zero cells from each matrix individually.
Secondly, we perform a Cartesian product on these filtered cells. For every pair of products obtained from this process, we calculate the corresponding linear transformation vector (Vab).
Subsequently, we count the number of pairs sharing identical transformation vectors – which also represents the total number of overlapping zones between the images.
Solution is coming soon for second method ……
Other Leet code Problem :