Leetcode 229. Majority Element II:Hey there, coding enthusiasts! Welcome back to another exciting coding session. Today’s problem is a treat—literally! We’re going to solve the “Majority Element II” or “LeetCode 229. ‘
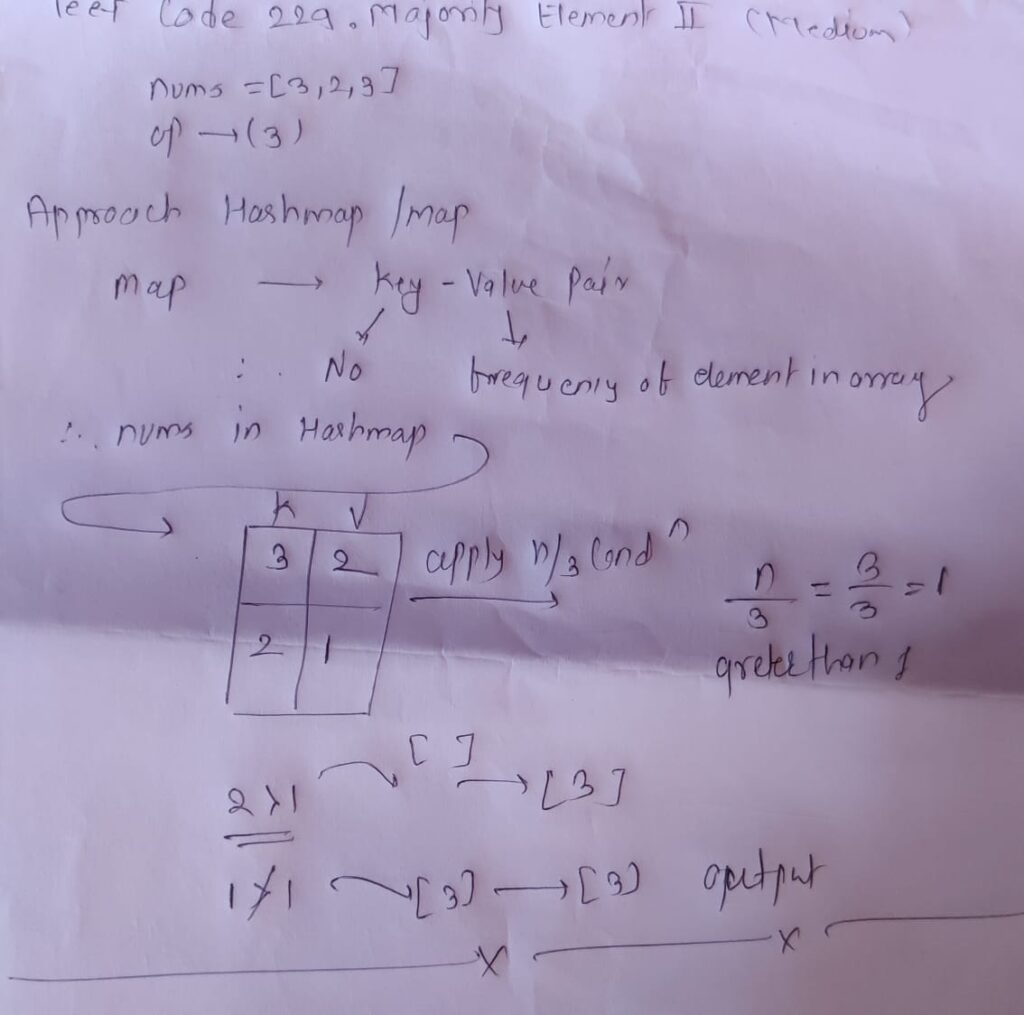
Method : HashMap Approach – Majority Element II
1: Initialize Data Structures
- Begin by creating the necessary data structures such as vectors (C++), lists (Java), arrays (Python), or objects (JavaScript) to store your results.
2: Count Element Occurrences
- Utilize data structures like unordered_map (C++), HashMap (Java), Counter (Python), or Map (JavaScript) to count the occurrences/ Frequency of each element in the input array.
3: Calculate the Threshold
- Determine the threshold, which is typically one-third of the array’s length. This threshold helps identify majority elements.
4: Iterate Over Counts
- Iterate through the count data structure, checking the occurrence of each element.
5: Identify Majority Elements
- If an element’s count surpasses the threshold, consider it a majority element and add it to the result.
6: Return the Result
- Finally, return the result, containing all identified majority elements in the array.
Codes : HashMap Approach – Majority Element II
C++ : Majority Element II
#include <vector>
#include <unordered_map>
class Solution {
public:
std::vector<int> majorityElement(std::vector<int>& nums) {
std::unordered_map<int, int> m;
for (int i = 0; i < nums.size(); i++) {
m[nums[i]]++;
}
int threshold = nums.size() / 3;
std::vector<int> result;
for (auto& it : m) {
int x = it.second;
if (x > threshold) {
result.push_back(it.first);
}
}
return result;
}
};
Java: Leet code 229
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Solution {
public List<Integer> majorityElement(int[] nums) {
Map<Integer, Integer> map = new HashMap<>();
for (int num : nums) {
map.put(num, map.getOrDefault(num, 0) + 1);
}
int threshold = nums.length / 3;
List<Integer> result = new ArrayList<>();
for (Map.Entry<Integer, Integer> entry : map.entrySet()) {
int count = entry.getValue();
if (count > threshold) {
result.add(entry.getKey());
}
}
return result;
}
}
Python: Leet Code 229
from collections import Counter
class Solution:
def majorityElement(self, nums):
counts = Counter(nums)
threshold = len(nums) // 3
result = []
for num, count in counts.items():
if count > threshold:
result.append(num)
return result
JavaScript: Majority Element II
/**
* @param {number[]} nums
* @return {number[]}
*/
var majorityElement = function(nums) {
const counts = new Map();
for (let num of nums) {
counts.set(num, (counts.get(num) || 0) + 1);
}
const threshold = Math.floor(nums.length / 3);
const result = [];
for (let [num, count] of counts.entries()) {
if (count > threshold) {
result.push(num);
}
}
return result;
};
Result Analysis Leet code 229
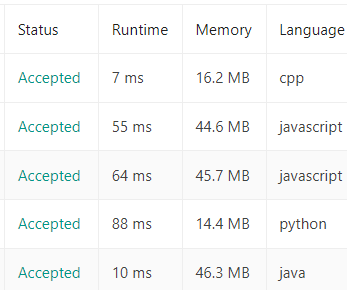
List of Some important Leet code Questions:
- Leet Code 2612 Minimum Reverse Operations
- Leet code 206 Reverse Linked List
- Leet Code 420 Strong Password Checker
- Leetcode 1359 Count All Valid Pickup and Delivery
- Leet code 799. Champagne Tower
- LeetCode 389. Find The Difference
- Leetcode 775. Find The Global and Local Inversions
- Leetcode 316. Remove Duplicate Letters
- LeetCode 2233 Maximum Product After K Increments
- LeetCode 880. Decoded String at Index
- LeetCode 905. Sort Array By Parity
- LeetCode 896. Monotonic Array
- LeetCode 132. Pattern